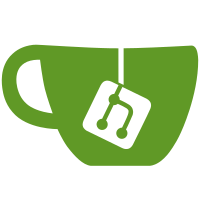
DisplayDevices can be released when DisplayManager removes them from the display list, or (for virtual displays) when the surface is set to NULL. We were only cleaning up HWC resources associated with the display in the first case. Bug: 8384764 Change-Id: Id3d226dd7178fbe6d0a2ac4e2660b864ee073de3
218 lines
6.6 KiB
C++
218 lines
6.6 KiB
C++
/*
|
|
* Copyright (C) 2007 The Android Open Source Project
|
|
*
|
|
* Licensed under the Apache License, Version 2.0 (the "License");
|
|
* you may not use this file except in compliance with the License.
|
|
* You may obtain a copy of the License at
|
|
*
|
|
* http://www.apache.org/licenses/LICENSE-2.0
|
|
*
|
|
* Unless required by applicable law or agreed to in writing, software
|
|
* distributed under the License is distributed on an "AS IS" BASIS,
|
|
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
* See the License for the specific language governing permissions and
|
|
* limitations under the License.
|
|
*/
|
|
|
|
#ifndef ANDROID_DISPLAY_DEVICE_H
|
|
#define ANDROID_DISPLAY_DEVICE_H
|
|
|
|
#include <stdlib.h>
|
|
|
|
#include <ui/PixelFormat.h>
|
|
#include <ui/Region.h>
|
|
|
|
#include <EGL/egl.h>
|
|
#include <EGL/eglext.h>
|
|
|
|
#include <utils/Mutex.h>
|
|
#include <utils/Timers.h>
|
|
|
|
#include <hardware/hwcomposer_defs.h>
|
|
|
|
#include "Transform.h"
|
|
|
|
struct ANativeWindow;
|
|
|
|
namespace android {
|
|
|
|
class DisplayInfo;
|
|
class DisplaySurface;
|
|
class Layer;
|
|
class SurfaceFlinger;
|
|
class HWComposer;
|
|
|
|
class DisplayDevice : public LightRefBase<DisplayDevice>
|
|
{
|
|
public:
|
|
// region in layer-stack space
|
|
mutable Region dirtyRegion;
|
|
// region in screen space
|
|
mutable Region swapRegion;
|
|
// region in screen space
|
|
Region undefinedRegion;
|
|
|
|
enum DisplayType {
|
|
DISPLAY_ID_INVALID = -1,
|
|
DISPLAY_PRIMARY = HWC_DISPLAY_PRIMARY,
|
|
DISPLAY_EXTERNAL = HWC_DISPLAY_EXTERNAL,
|
|
NUM_DISPLAY_TYPES = HWC_NUM_DISPLAY_TYPES,
|
|
DISPLAY_VIRTUAL = HWC_NUM_DISPLAY_TYPES
|
|
};
|
|
|
|
enum {
|
|
PARTIAL_UPDATES = 0x00020000, // video driver feature
|
|
SWAP_RECTANGLE = 0x00080000,
|
|
};
|
|
|
|
enum {
|
|
NO_LAYER_STACK = 0xFFFFFFFF,
|
|
};
|
|
|
|
DisplayDevice(
|
|
const sp<SurfaceFlinger>& flinger,
|
|
DisplayType type,
|
|
int32_t hwcId, // negative for non-HWC-composited displays
|
|
bool isSecure,
|
|
const wp<IBinder>& displayToken,
|
|
const sp<DisplaySurface>& displaySurface,
|
|
EGLConfig config);
|
|
|
|
~DisplayDevice();
|
|
|
|
// whether this is a valid object. An invalid DisplayDevice is returned
|
|
// when an non existing id is requested
|
|
bool isValid() const;
|
|
|
|
// isSecure indicates whether this display can be trusted to display
|
|
// secure surfaces.
|
|
bool isSecure() const { return mIsSecure; }
|
|
|
|
// Flip the front and back buffers if the back buffer is "dirty". Might
|
|
// be instantaneous, might involve copying the frame buffer around.
|
|
void flip(const Region& dirty) const;
|
|
|
|
int getWidth() const;
|
|
int getHeight() const;
|
|
PixelFormat getFormat() const;
|
|
uint32_t getFlags() const;
|
|
|
|
EGLSurface getEGLSurface() const;
|
|
|
|
void setVisibleLayersSortedByZ(const Vector< sp<Layer> >& layers);
|
|
const Vector< sp<Layer> >& getVisibleLayersSortedByZ() const;
|
|
bool getSecureLayerVisible() const;
|
|
Region getDirtyRegion(bool repaintEverything) const;
|
|
|
|
void setLayerStack(uint32_t stack);
|
|
void setProjection(int orientation, const Rect& viewport, const Rect& frame);
|
|
|
|
int getOrientation() const { return mOrientation; }
|
|
const Transform& getTransform() const { return mGlobalTransform; }
|
|
const Rect getViewport() const { return mViewport; }
|
|
const Rect getFrame() const { return mFrame; }
|
|
const Rect& getScissor() const { return mScissor; }
|
|
bool needsFiltering() const { return mNeedsFiltering; }
|
|
|
|
uint32_t getLayerStack() const { return mLayerStack; }
|
|
int32_t getDisplayType() const { return mType; }
|
|
int32_t getHwcDisplayId() const { return mHwcDisplayId; }
|
|
const wp<IBinder>& getDisplayToken() const { return mDisplayToken; }
|
|
|
|
void swapBuffers(HWComposer& hwc) const;
|
|
status_t compositionComplete() const;
|
|
|
|
// called after h/w composer has completed its set() call
|
|
void onSwapBuffersCompleted(HWComposer& hwc) const;
|
|
|
|
Rect getBounds() const {
|
|
return Rect(mDisplayWidth, mDisplayHeight);
|
|
}
|
|
inline Rect bounds() const { return getBounds(); }
|
|
|
|
void setDisplayName(const String8& displayName);
|
|
const String8& getDisplayName() const { return mDisplayName; }
|
|
|
|
static EGLBoolean makeCurrent(EGLDisplay dpy,
|
|
const sp<const DisplayDevice>& hw, EGLContext ctx);
|
|
|
|
static void setViewportAndProjection(const sp<const DisplayDevice>& hw);
|
|
|
|
/* ------------------------------------------------------------------------
|
|
* blank / unblank management
|
|
*/
|
|
void releaseScreen() const;
|
|
void acquireScreen() const;
|
|
bool isScreenAcquired() const;
|
|
bool canDraw() const;
|
|
|
|
// release HWC resources (if any) for removable displays
|
|
void disconnect(HWComposer& hwc);
|
|
|
|
/* ------------------------------------------------------------------------
|
|
* Debugging
|
|
*/
|
|
uint32_t getPageFlipCount() const;
|
|
void dump(String8& result, char* buffer, size_t SIZE) const;
|
|
|
|
private:
|
|
/*
|
|
* Constants, set during initialization
|
|
*/
|
|
sp<SurfaceFlinger> mFlinger;
|
|
DisplayType mType;
|
|
int32_t mHwcDisplayId;
|
|
wp<IBinder> mDisplayToken;
|
|
|
|
// ANativeWindow this display is rendering into
|
|
sp<ANativeWindow> mNativeWindow;
|
|
sp<DisplaySurface> mDisplaySurface;
|
|
|
|
EGLDisplay mDisplay;
|
|
EGLSurface mSurface;
|
|
EGLContext mContext;
|
|
int mDisplayWidth;
|
|
int mDisplayHeight;
|
|
PixelFormat mFormat;
|
|
uint32_t mFlags;
|
|
mutable uint32_t mPageFlipCount;
|
|
String8 mDisplayName;
|
|
bool mIsSecure;
|
|
|
|
/*
|
|
* Can only accessed from the main thread, these members
|
|
* don't need synchronization.
|
|
*/
|
|
|
|
// list of visible layers on that display
|
|
Vector< sp<Layer> > mVisibleLayersSortedByZ;
|
|
|
|
// Whether we have a visible secure layer on this display
|
|
bool mSecureLayerVisible;
|
|
|
|
// Whether the screen is blanked;
|
|
mutable int mScreenAcquired;
|
|
|
|
|
|
/*
|
|
* Transaction state
|
|
*/
|
|
static status_t orientationToTransfrom(int orientation,
|
|
int w, int h, Transform* tr);
|
|
|
|
uint32_t mLayerStack;
|
|
int mOrientation;
|
|
// user-provided visible area of the layer stack
|
|
Rect mViewport;
|
|
// user-provided rectangle where mViewport gets mapped to
|
|
Rect mFrame;
|
|
// pre-computed scissor to apply to the display
|
|
Rect mScissor;
|
|
Transform mGlobalTransform;
|
|
bool mNeedsFiltering;
|
|
};
|
|
|
|
}; // namespace android
|
|
|
|
#endif // ANDROID_DISPLAY_DEVICE_H
|