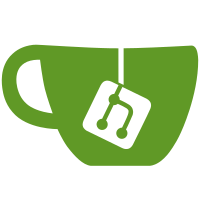
This adds an allocateBuffers method to BufferQueue, which instructs it to allocate up to the maximum number of buffers allowed by the current configuration. The goal is that this method can be called ahead of render time, which will prevent dequeueBuffers from blocking in allocation and inducing jank. This interface is also plumbed up to the native Surface (and, in another change, up to the Java Surface and ThreadedRenderer). Bug: 11792166 Change-Id: I4aa96b4351ea1c95ed5db228ca3ef98303229c74
66 lines
2.4 KiB
C++
66 lines
2.4 KiB
C++
/*
|
|
* Copyright 2014 The Android Open Source Project
|
|
*
|
|
* Licensed under the Apache License, Version 2.0 (the "License");
|
|
* you may not use this file except in compliance with the License.
|
|
* You may obtain a copy of the License at
|
|
*
|
|
* http://www.apache.org/licenses/LICENSE-2.0
|
|
*
|
|
* Unless required by applicable law or agreed to in writing, software
|
|
* distributed under the License is distributed on an "AS IS" BASIS,
|
|
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
* See the License for the specific language governing permissions and
|
|
* limitations under the License.
|
|
*/
|
|
|
|
#ifndef ANDROID_MONITORED_PRODUCER_H
|
|
#define ANDROID_MONITORED_PRODUCER_H
|
|
|
|
#include <gui/IGraphicBufferProducer.h>
|
|
|
|
namespace android {
|
|
|
|
class IProducerListener;
|
|
class NativeHandle;
|
|
class SurfaceFlinger;
|
|
|
|
// MonitoredProducer wraps an IGraphicBufferProducer so that SurfaceFlinger will
|
|
// be notified upon its destruction
|
|
class MonitoredProducer : public IGraphicBufferProducer {
|
|
public:
|
|
MonitoredProducer(const sp<IGraphicBufferProducer>& producer,
|
|
const sp<SurfaceFlinger>& flinger);
|
|
virtual ~MonitoredProducer();
|
|
|
|
// From IGraphicBufferProducer
|
|
virtual status_t requestBuffer(int slot, sp<GraphicBuffer>* buf);
|
|
virtual status_t setBufferCount(int bufferCount);
|
|
virtual status_t dequeueBuffer(int* slot, sp<Fence>* fence, bool async,
|
|
uint32_t w, uint32_t h, uint32_t format, uint32_t usage);
|
|
virtual status_t detachBuffer(int slot);
|
|
virtual status_t detachNextBuffer(sp<GraphicBuffer>* outBuffer,
|
|
sp<Fence>* outFence);
|
|
virtual status_t attachBuffer(int* outSlot,
|
|
const sp<GraphicBuffer>& buffer);
|
|
virtual status_t queueBuffer(int slot, const QueueBufferInput& input,
|
|
QueueBufferOutput* output);
|
|
virtual void cancelBuffer(int slot, const sp<Fence>& fence);
|
|
virtual int query(int what, int* value);
|
|
virtual status_t connect(const sp<IProducerListener>& token, int api,
|
|
bool producerControlledByApp, QueueBufferOutput* output);
|
|
virtual status_t disconnect(int api);
|
|
virtual status_t setSidebandStream(const sp<NativeHandle>& stream);
|
|
virtual void allocateBuffers(bool async, uint32_t width, uint32_t height,
|
|
uint32_t format, uint32_t usage);
|
|
virtual IBinder* onAsBinder();
|
|
|
|
private:
|
|
sp<IGraphicBufferProducer> mProducer;
|
|
sp<SurfaceFlinger> mFlinger;
|
|
};
|
|
|
|
}; // namespace android
|
|
|
|
#endif // ANDROID_MONITORED_PRODUCER_H
|