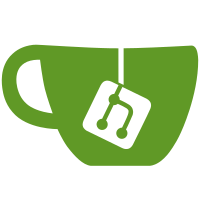
Use Vendor ID, Product ID and optionally the Version to locate keymaps and configuration files for external devices. Moved virtual key definition parsing to native code so that EventHub can identify touch screens with virtual keys and load the appropriate key layout file. Cleaned up a lot of old code in EventHub. Fixed a regression in ViewRoot's fallback event handling. Fixed a minor bug in FileMap that caused it to try to munmap or close invalid handled when released if the attempt to map the file failed. Added a couple of new String8 conveniences for formatting strings. Modified Tokenizer to fall back to open+read when mmap fails since we can't mmap sysfs files as needed to open the virtual key definition files in /sys/board_properties/. Change-Id: I6ca5e5f9547619fd082ddac47e87ce185da69ee6
1093 lines
36 KiB
C++
1093 lines
36 KiB
C++
//
|
|
// Copyright 2005 The Android Open Source Project
|
|
//
|
|
// Handle events, like key input and vsync.
|
|
//
|
|
// The goal is to provide an optimized solution for Linux, not an
|
|
// implementation that works well across all platforms. We expect
|
|
// events to arrive on file descriptors, so that we can use a select()
|
|
// select() call to sleep.
|
|
//
|
|
// We can't select() on anything but network sockets in Windows, so we
|
|
// provide an alternative implementation of waitEvent for that platform.
|
|
//
|
|
#define LOG_TAG "EventHub"
|
|
|
|
//#define LOG_NDEBUG 0
|
|
|
|
#include <ui/EventHub.h>
|
|
#include <hardware_legacy/power.h>
|
|
|
|
#include <cutils/properties.h>
|
|
#include <utils/Log.h>
|
|
#include <utils/Timers.h>
|
|
#include <utils/threads.h>
|
|
#include <utils/Errors.h>
|
|
|
|
#include <stdlib.h>
|
|
#include <stdio.h>
|
|
#include <unistd.h>
|
|
#include <fcntl.h>
|
|
#include <memory.h>
|
|
#include <errno.h>
|
|
#include <assert.h>
|
|
|
|
#include <ui/KeyLayoutMap.h>
|
|
#include <ui/KeyCharacterMap.h>
|
|
#include <ui/VirtualKeyMap.h>
|
|
|
|
#include <string.h>
|
|
#include <stdint.h>
|
|
#include <dirent.h>
|
|
#ifdef HAVE_INOTIFY
|
|
# include <sys/inotify.h>
|
|
#endif
|
|
#ifdef HAVE_ANDROID_OS
|
|
# include <sys/limits.h> /* not part of Linux */
|
|
#endif
|
|
#include <sys/poll.h>
|
|
#include <sys/ioctl.h>
|
|
|
|
/* this macro is used to tell if "bit" is set in "array"
|
|
* it selects a byte from the array, and does a boolean AND
|
|
* operation with a byte that only has the relevant bit set.
|
|
* eg. to check for the 12th bit, we do (array[1] & 1<<4)
|
|
*/
|
|
#define test_bit(bit, array) (array[bit/8] & (1<<(bit%8)))
|
|
|
|
/* this macro computes the number of bytes needed to represent a bit array of the specified size */
|
|
#define sizeof_bit_array(bits) ((bits + 7) / 8)
|
|
|
|
#ifndef ABS_MT_TOUCH_MAJOR
|
|
#define ABS_MT_TOUCH_MAJOR 0x30 /* Major axis of touching ellipse */
|
|
#endif
|
|
|
|
#ifndef ABS_MT_POSITION_X
|
|
#define ABS_MT_POSITION_X 0x35 /* Center X ellipse position */
|
|
#endif
|
|
|
|
#ifndef ABS_MT_POSITION_Y
|
|
#define ABS_MT_POSITION_Y 0x36 /* Center Y ellipse position */
|
|
#endif
|
|
|
|
// Fd at index 0 is always reserved for inotify
|
|
#define FIRST_ACTUAL_DEVICE_INDEX 1
|
|
|
|
#define INDENT " "
|
|
#define INDENT2 " "
|
|
#define INDENT3 " "
|
|
|
|
namespace android {
|
|
|
|
static const char *WAKE_LOCK_ID = "KeyEvents";
|
|
static const char *DEVICE_PATH = "/dev/input";
|
|
|
|
/* return the larger integer */
|
|
static inline int max(int v1, int v2)
|
|
{
|
|
return (v1 > v2) ? v1 : v2;
|
|
}
|
|
|
|
static inline const char* toString(bool value) {
|
|
return value ? "true" : "false";
|
|
}
|
|
|
|
// --- EventHub::Device ---
|
|
|
|
EventHub::Device::Device(int fd, int32_t id, const String8& path,
|
|
const InputDeviceIdentifier& identifier) :
|
|
next(NULL),
|
|
fd(fd), id(id), path(path), identifier(identifier),
|
|
classes(0), keyBitmask(NULL), configuration(NULL), virtualKeyMap(NULL) {
|
|
}
|
|
|
|
EventHub::Device::~Device() {
|
|
close();
|
|
delete[] keyBitmask;
|
|
delete configuration;
|
|
delete virtualKeyMap;
|
|
}
|
|
|
|
void EventHub::Device::close() {
|
|
if (fd >= 0) {
|
|
::close(fd);
|
|
fd = -1;
|
|
}
|
|
}
|
|
|
|
|
|
// --- EventHub ---
|
|
|
|
EventHub::EventHub(void) :
|
|
mError(NO_INIT), mBuiltInKeyboardId(-1), mNextDeviceId(1),
|
|
mOpeningDevices(0), mClosingDevices(0),
|
|
mOpened(false), mNeedToSendFinishedDeviceScan(false),
|
|
mInputBufferIndex(0), mInputBufferCount(0), mInputFdIndex(0) {
|
|
acquire_wake_lock(PARTIAL_WAKE_LOCK, WAKE_LOCK_ID);
|
|
#ifdef EV_SW
|
|
memset(mSwitches, 0, sizeof(mSwitches));
|
|
#endif
|
|
}
|
|
|
|
EventHub::~EventHub(void) {
|
|
release_wake_lock(WAKE_LOCK_ID);
|
|
// we should free stuff here...
|
|
}
|
|
|
|
status_t EventHub::errorCheck() const {
|
|
return mError;
|
|
}
|
|
|
|
String8 EventHub::getDeviceName(int32_t deviceId) const {
|
|
AutoMutex _l(mLock);
|
|
Device* device = getDeviceLocked(deviceId);
|
|
if (device == NULL) return String8();
|
|
return device->identifier.name;
|
|
}
|
|
|
|
uint32_t EventHub::getDeviceClasses(int32_t deviceId) const {
|
|
AutoMutex _l(mLock);
|
|
Device* device = getDeviceLocked(deviceId);
|
|
if (device == NULL) return 0;
|
|
return device->classes;
|
|
}
|
|
|
|
void EventHub::getConfiguration(int32_t deviceId, PropertyMap* outConfiguration) const {
|
|
AutoMutex _l(mLock);
|
|
Device* device = getDeviceLocked(deviceId);
|
|
if (device && device->configuration) {
|
|
*outConfiguration = *device->configuration;
|
|
} else {
|
|
outConfiguration->clear();
|
|
}
|
|
}
|
|
|
|
status_t EventHub::getAbsoluteAxisInfo(int32_t deviceId, int axis,
|
|
RawAbsoluteAxisInfo* outAxisInfo) const {
|
|
outAxisInfo->clear();
|
|
|
|
AutoMutex _l(mLock);
|
|
Device* device = getDeviceLocked(deviceId);
|
|
if (device == NULL) return -1;
|
|
|
|
struct input_absinfo info;
|
|
|
|
if(ioctl(device->fd, EVIOCGABS(axis), &info)) {
|
|
LOGW("Error reading absolute controller %d for device %s fd %d\n",
|
|
axis, device->identifier.name.string(), device->fd);
|
|
return -errno;
|
|
}
|
|
|
|
if (info.minimum != info.maximum) {
|
|
outAxisInfo->valid = true;
|
|
outAxisInfo->minValue = info.minimum;
|
|
outAxisInfo->maxValue = info.maximum;
|
|
outAxisInfo->flat = info.flat;
|
|
outAxisInfo->fuzz = info.fuzz;
|
|
}
|
|
return OK;
|
|
}
|
|
|
|
int32_t EventHub::getScanCodeState(int32_t deviceId, int32_t scanCode) const {
|
|
if (scanCode >= 0 && scanCode <= KEY_MAX) {
|
|
AutoMutex _l(mLock);
|
|
|
|
Device* device = getDeviceLocked(deviceId);
|
|
if (device != NULL) {
|
|
return getScanCodeStateLocked(device, scanCode);
|
|
}
|
|
}
|
|
return AKEY_STATE_UNKNOWN;
|
|
}
|
|
|
|
int32_t EventHub::getScanCodeStateLocked(Device* device, int32_t scanCode) const {
|
|
uint8_t key_bitmask[sizeof_bit_array(KEY_MAX + 1)];
|
|
memset(key_bitmask, 0, sizeof(key_bitmask));
|
|
if (ioctl(device->fd,
|
|
EVIOCGKEY(sizeof(key_bitmask)), key_bitmask) >= 0) {
|
|
return test_bit(scanCode, key_bitmask) ? AKEY_STATE_DOWN : AKEY_STATE_UP;
|
|
}
|
|
return AKEY_STATE_UNKNOWN;
|
|
}
|
|
|
|
int32_t EventHub::getKeyCodeState(int32_t deviceId, int32_t keyCode) const {
|
|
AutoMutex _l(mLock);
|
|
|
|
Device* device = getDeviceLocked(deviceId);
|
|
if (device != NULL) {
|
|
return getKeyCodeStateLocked(device, keyCode);
|
|
}
|
|
return AKEY_STATE_UNKNOWN;
|
|
}
|
|
|
|
int32_t EventHub::getKeyCodeStateLocked(Device* device, int32_t keyCode) const {
|
|
if (!device->keyMap.haveKeyLayout()) {
|
|
return AKEY_STATE_UNKNOWN;
|
|
}
|
|
|
|
Vector<int32_t> scanCodes;
|
|
device->keyMap.keyLayoutMap->findScanCodes(keyCode, &scanCodes);
|
|
|
|
uint8_t key_bitmask[sizeof_bit_array(KEY_MAX + 1)];
|
|
memset(key_bitmask, 0, sizeof(key_bitmask));
|
|
if (ioctl(device->fd, EVIOCGKEY(sizeof(key_bitmask)), key_bitmask) >= 0) {
|
|
#if 0
|
|
for (size_t i=0; i<=KEY_MAX; i++) {
|
|
LOGI("(Scan code %d: down=%d)", i, test_bit(i, key_bitmask));
|
|
}
|
|
#endif
|
|
const size_t N = scanCodes.size();
|
|
for (size_t i=0; i<N && i<=KEY_MAX; i++) {
|
|
int32_t sc = scanCodes.itemAt(i);
|
|
//LOGI("Code %d: down=%d", sc, test_bit(sc, key_bitmask));
|
|
if (sc >= 0 && sc <= KEY_MAX && test_bit(sc, key_bitmask)) {
|
|
return AKEY_STATE_DOWN;
|
|
}
|
|
}
|
|
return AKEY_STATE_UP;
|
|
}
|
|
return AKEY_STATE_UNKNOWN;
|
|
}
|
|
|
|
int32_t EventHub::getSwitchState(int32_t deviceId, int32_t sw) const {
|
|
#ifdef EV_SW
|
|
if (sw >= 0 && sw <= SW_MAX) {
|
|
AutoMutex _l(mLock);
|
|
|
|
Device* device = getDeviceLocked(deviceId);
|
|
if (device != NULL) {
|
|
return getSwitchStateLocked(device, sw);
|
|
}
|
|
}
|
|
#endif
|
|
return AKEY_STATE_UNKNOWN;
|
|
}
|
|
|
|
int32_t EventHub::getSwitchStateLocked(Device* device, int32_t sw) const {
|
|
uint8_t sw_bitmask[sizeof_bit_array(SW_MAX + 1)];
|
|
memset(sw_bitmask, 0, sizeof(sw_bitmask));
|
|
if (ioctl(device->fd,
|
|
EVIOCGSW(sizeof(sw_bitmask)), sw_bitmask) >= 0) {
|
|
return test_bit(sw, sw_bitmask) ? AKEY_STATE_DOWN : AKEY_STATE_UP;
|
|
}
|
|
return AKEY_STATE_UNKNOWN;
|
|
}
|
|
|
|
bool EventHub::markSupportedKeyCodes(int32_t deviceId, size_t numCodes,
|
|
const int32_t* keyCodes, uint8_t* outFlags) const {
|
|
AutoMutex _l(mLock);
|
|
|
|
Device* device = getDeviceLocked(deviceId);
|
|
if (device != NULL) {
|
|
return markSupportedKeyCodesLocked(device, numCodes, keyCodes, outFlags);
|
|
}
|
|
return false;
|
|
}
|
|
|
|
bool EventHub::markSupportedKeyCodesLocked(Device* device, size_t numCodes,
|
|
const int32_t* keyCodes, uint8_t* outFlags) const {
|
|
if (!device->keyMap.haveKeyLayout() || !device->keyBitmask) {
|
|
return false;
|
|
}
|
|
|
|
Vector<int32_t> scanCodes;
|
|
for (size_t codeIndex = 0; codeIndex < numCodes; codeIndex++) {
|
|
scanCodes.clear();
|
|
|
|
status_t err = device->keyMap.keyLayoutMap->findScanCodes(keyCodes[codeIndex], &scanCodes);
|
|
if (! err) {
|
|
// check the possible scan codes identified by the layout map against the
|
|
// map of codes actually emitted by the driver
|
|
for (size_t sc = 0; sc < scanCodes.size(); sc++) {
|
|
if (test_bit(scanCodes[sc], device->keyBitmask)) {
|
|
outFlags[codeIndex] = 1;
|
|
break;
|
|
}
|
|
}
|
|
}
|
|
}
|
|
return true;
|
|
}
|
|
|
|
status_t EventHub::scancodeToKeycode(int32_t deviceId, int scancode,
|
|
int32_t* outKeycode, uint32_t* outFlags) const
|
|
{
|
|
AutoMutex _l(mLock);
|
|
Device* device = getDeviceLocked(deviceId);
|
|
|
|
if (device && device->keyMap.haveKeyLayout()) {
|
|
status_t err = device->keyMap.keyLayoutMap->map(scancode, outKeycode, outFlags);
|
|
if (err == NO_ERROR) {
|
|
return NO_ERROR;
|
|
}
|
|
}
|
|
|
|
if (mBuiltInKeyboardId != -1) {
|
|
device = getDeviceLocked(mBuiltInKeyboardId);
|
|
|
|
if (device && device->keyMap.haveKeyLayout()) {
|
|
status_t err = device->keyMap.keyLayoutMap->map(scancode, outKeycode, outFlags);
|
|
if (err == NO_ERROR) {
|
|
return NO_ERROR;
|
|
}
|
|
}
|
|
}
|
|
|
|
*outKeycode = 0;
|
|
*outFlags = 0;
|
|
return NAME_NOT_FOUND;
|
|
}
|
|
|
|
void EventHub::addExcludedDevice(const char* deviceName)
|
|
{
|
|
AutoMutex _l(mLock);
|
|
|
|
String8 name(deviceName);
|
|
mExcludedDevices.push_back(name);
|
|
}
|
|
|
|
bool EventHub::hasLed(int32_t deviceId, int32_t led) const {
|
|
AutoMutex _l(mLock);
|
|
Device* device = getDeviceLocked(deviceId);
|
|
if (device) {
|
|
uint8_t bitmask[sizeof_bit_array(LED_MAX + 1)];
|
|
memset(bitmask, 0, sizeof(bitmask));
|
|
if (ioctl(device->fd, EVIOCGBIT(EV_LED, sizeof(bitmask)), bitmask) >= 0) {
|
|
if (test_bit(led, bitmask)) {
|
|
return true;
|
|
}
|
|
}
|
|
}
|
|
return false;
|
|
}
|
|
|
|
void EventHub::setLedState(int32_t deviceId, int32_t led, bool on) {
|
|
AutoMutex _l(mLock);
|
|
Device* device = getDeviceLocked(deviceId);
|
|
if (device) {
|
|
struct input_event ev;
|
|
ev.time.tv_sec = 0;
|
|
ev.time.tv_usec = 0;
|
|
ev.type = EV_LED;
|
|
ev.code = led;
|
|
ev.value = on ? 1 : 0;
|
|
|
|
ssize_t nWrite;
|
|
do {
|
|
nWrite = write(device->fd, &ev, sizeof(struct input_event));
|
|
} while (nWrite == -1 && errno == EINTR);
|
|
}
|
|
}
|
|
|
|
void EventHub::getVirtualKeyDefinitions(int32_t deviceId,
|
|
Vector<VirtualKeyDefinition>& outVirtualKeys) const {
|
|
outVirtualKeys.clear();
|
|
|
|
AutoMutex _l(mLock);
|
|
Device* device = getDeviceLocked(deviceId);
|
|
if (device && device->virtualKeyMap) {
|
|
outVirtualKeys.appendVector(device->virtualKeyMap->getVirtualKeys());
|
|
}
|
|
}
|
|
|
|
EventHub::Device* EventHub::getDeviceLocked(int32_t deviceId) const {
|
|
if (deviceId == 0) {
|
|
deviceId = mBuiltInKeyboardId;
|
|
}
|
|
|
|
size_t numDevices = mDevices.size();
|
|
for (size_t i = FIRST_ACTUAL_DEVICE_INDEX; i < numDevices; i++) {
|
|
Device* device = mDevices[i];
|
|
if (device->id == deviceId) {
|
|
return device;
|
|
}
|
|
}
|
|
return NULL;
|
|
}
|
|
|
|
bool EventHub::getEvent(RawEvent* outEvent) {
|
|
outEvent->deviceId = 0;
|
|
outEvent->type = 0;
|
|
outEvent->scanCode = 0;
|
|
outEvent->keyCode = 0;
|
|
outEvent->flags = 0;
|
|
outEvent->value = 0;
|
|
outEvent->when = 0;
|
|
|
|
// Note that we only allow one caller to getEvent(), so don't need
|
|
// to do locking here... only when adding/removing devices.
|
|
|
|
if (!mOpened) {
|
|
mError = openPlatformInput() ? NO_ERROR : UNKNOWN_ERROR;
|
|
mOpened = true;
|
|
mNeedToSendFinishedDeviceScan = true;
|
|
}
|
|
|
|
for (;;) {
|
|
// Report any devices that had last been added/removed.
|
|
if (mClosingDevices != NULL) {
|
|
Device* device = mClosingDevices;
|
|
LOGV("Reporting device closed: id=%d, name=%s\n",
|
|
device->id, device->path.string());
|
|
mClosingDevices = device->next;
|
|
if (device->id == mBuiltInKeyboardId) {
|
|
outEvent->deviceId = 0;
|
|
} else {
|
|
outEvent->deviceId = device->id;
|
|
}
|
|
outEvent->type = DEVICE_REMOVED;
|
|
outEvent->when = systemTime(SYSTEM_TIME_MONOTONIC);
|
|
delete device;
|
|
mNeedToSendFinishedDeviceScan = true;
|
|
return true;
|
|
}
|
|
|
|
if (mOpeningDevices != NULL) {
|
|
Device* device = mOpeningDevices;
|
|
LOGV("Reporting device opened: id=%d, name=%s\n",
|
|
device->id, device->path.string());
|
|
mOpeningDevices = device->next;
|
|
if (device->id == mBuiltInKeyboardId) {
|
|
outEvent->deviceId = 0;
|
|
} else {
|
|
outEvent->deviceId = device->id;
|
|
}
|
|
outEvent->type = DEVICE_ADDED;
|
|
outEvent->when = systemTime(SYSTEM_TIME_MONOTONIC);
|
|
mNeedToSendFinishedDeviceScan = true;
|
|
return true;
|
|
}
|
|
|
|
if (mNeedToSendFinishedDeviceScan) {
|
|
mNeedToSendFinishedDeviceScan = false;
|
|
outEvent->type = FINISHED_DEVICE_SCAN;
|
|
outEvent->when = systemTime(SYSTEM_TIME_MONOTONIC);
|
|
return true;
|
|
}
|
|
|
|
// Grab the next input event.
|
|
for (;;) {
|
|
// Consume buffered input events, if any.
|
|
if (mInputBufferIndex < mInputBufferCount) {
|
|
const struct input_event& iev = mInputBufferData[mInputBufferIndex++];
|
|
const Device* device = mDevices[mInputFdIndex];
|
|
|
|
LOGV("%s got: t0=%d, t1=%d, type=%d, code=%d, v=%d", device->path.string(),
|
|
(int) iev.time.tv_sec, (int) iev.time.tv_usec, iev.type, iev.code, iev.value);
|
|
if (device->id == mBuiltInKeyboardId) {
|
|
outEvent->deviceId = 0;
|
|
} else {
|
|
outEvent->deviceId = device->id;
|
|
}
|
|
outEvent->type = iev.type;
|
|
outEvent->scanCode = iev.code;
|
|
outEvent->flags = 0;
|
|
if (iev.type == EV_KEY) {
|
|
outEvent->keyCode = AKEYCODE_UNKNOWN;
|
|
if (device->keyMap.haveKeyLayout()) {
|
|
status_t err = device->keyMap.keyLayoutMap->map(iev.code,
|
|
&outEvent->keyCode, &outEvent->flags);
|
|
LOGV("iev.code=%d keyCode=%d flags=0x%08x err=%d\n",
|
|
iev.code, outEvent->keyCode, outEvent->flags, err);
|
|
}
|
|
} else {
|
|
outEvent->keyCode = iev.code;
|
|
}
|
|
outEvent->value = iev.value;
|
|
|
|
// Use an event timestamp in the same timebase as
|
|
// java.lang.System.nanoTime() and android.os.SystemClock.uptimeMillis()
|
|
// as expected by the rest of the system.
|
|
outEvent->when = systemTime(SYSTEM_TIME_MONOTONIC);
|
|
return true;
|
|
}
|
|
|
|
// Finish reading all events from devices identified in previous poll().
|
|
// This code assumes that mInputDeviceIndex is initially 0 and that the
|
|
// revents member of pollfd is initialized to 0 when the device is first added.
|
|
// Since mFds[0] is used for inotify, we process regular events starting at index 1.
|
|
mInputFdIndex += 1;
|
|
if (mInputFdIndex >= mFds.size()) {
|
|
break;
|
|
}
|
|
|
|
const struct pollfd& pfd = mFds[mInputFdIndex];
|
|
if (pfd.revents & POLLIN) {
|
|
int32_t readSize = read(pfd.fd, mInputBufferData,
|
|
sizeof(struct input_event) * INPUT_BUFFER_SIZE);
|
|
if (readSize < 0) {
|
|
if (errno != EAGAIN && errno != EINTR) {
|
|
LOGW("could not get event (errno=%d)", errno);
|
|
}
|
|
} else if ((readSize % sizeof(struct input_event)) != 0) {
|
|
LOGE("could not get event (wrong size: %d)", readSize);
|
|
} else {
|
|
mInputBufferCount = size_t(readSize) / sizeof(struct input_event);
|
|
mInputBufferIndex = 0;
|
|
}
|
|
}
|
|
}
|
|
|
|
#if HAVE_INOTIFY
|
|
// readNotify() will modify mFDs and mFDCount, so this must be done after
|
|
// processing all other events.
|
|
if(mFds[0].revents & POLLIN) {
|
|
readNotify(mFds[0].fd);
|
|
mFds.editItemAt(0).revents = 0;
|
|
continue; // report added or removed devices immediately
|
|
}
|
|
#endif
|
|
|
|
mInputFdIndex = 0;
|
|
|
|
// Poll for events. Mind the wake lock dance!
|
|
// We hold a wake lock at all times except during poll(). This works due to some
|
|
// subtle choreography. When a device driver has pending (unread) events, it acquires
|
|
// a kernel wake lock. However, once the last pending event has been read, the device
|
|
// driver will release the kernel wake lock. To prevent the system from going to sleep
|
|
// when this happens, the EventHub holds onto its own user wake lock while the client
|
|
// is processing events. Thus the system can only sleep if there are no events
|
|
// pending or currently being processed.
|
|
release_wake_lock(WAKE_LOCK_ID);
|
|
|
|
int pollResult = poll(mFds.editArray(), mFds.size(), -1);
|
|
|
|
acquire_wake_lock(PARTIAL_WAKE_LOCK, WAKE_LOCK_ID);
|
|
|
|
if (pollResult <= 0) {
|
|
if (errno != EINTR) {
|
|
LOGW("poll failed (errno=%d)\n", errno);
|
|
usleep(100000);
|
|
}
|
|
}
|
|
}
|
|
}
|
|
|
|
/*
|
|
* Open the platform-specific input device.
|
|
*/
|
|
bool EventHub::openPlatformInput(void) {
|
|
/*
|
|
* Open platform-specific input device(s).
|
|
*/
|
|
int res, fd;
|
|
|
|
#ifdef HAVE_INOTIFY
|
|
fd = inotify_init();
|
|
res = inotify_add_watch(fd, DEVICE_PATH, IN_DELETE | IN_CREATE);
|
|
if(res < 0) {
|
|
LOGE("could not add watch for %s, %s\n", DEVICE_PATH, strerror(errno));
|
|
}
|
|
#else
|
|
/*
|
|
* The code in EventHub::getEvent assumes that mFDs[0] is an inotify fd.
|
|
* We allocate space for it and set it to something invalid.
|
|
*/
|
|
fd = -1;
|
|
#endif
|
|
|
|
// Reserve fd index 0 for inotify.
|
|
struct pollfd pollfd;
|
|
pollfd.fd = fd;
|
|
pollfd.events = POLLIN;
|
|
pollfd.revents = 0;
|
|
mFds.push(pollfd);
|
|
mDevices.push(NULL);
|
|
|
|
res = scanDir(DEVICE_PATH);
|
|
if(res < 0) {
|
|
LOGE("scan dir failed for %s\n", DEVICE_PATH);
|
|
}
|
|
|
|
return true;
|
|
}
|
|
|
|
// ----------------------------------------------------------------------------
|
|
|
|
static bool containsNonZeroByte(const uint8_t* array, uint32_t startIndex, uint32_t endIndex) {
|
|
const uint8_t* end = array + endIndex;
|
|
array += startIndex;
|
|
while (array != end) {
|
|
if (*(array++) != 0) {
|
|
return true;
|
|
}
|
|
}
|
|
return false;
|
|
}
|
|
|
|
static const int32_t GAMEPAD_KEYCODES[] = {
|
|
AKEYCODE_BUTTON_A, AKEYCODE_BUTTON_B, AKEYCODE_BUTTON_C,
|
|
AKEYCODE_BUTTON_X, AKEYCODE_BUTTON_Y, AKEYCODE_BUTTON_Z,
|
|
AKEYCODE_BUTTON_L1, AKEYCODE_BUTTON_R1,
|
|
AKEYCODE_BUTTON_L2, AKEYCODE_BUTTON_R2,
|
|
AKEYCODE_BUTTON_THUMBL, AKEYCODE_BUTTON_THUMBR,
|
|
AKEYCODE_BUTTON_START, AKEYCODE_BUTTON_SELECT, AKEYCODE_BUTTON_MODE
|
|
};
|
|
|
|
int EventHub::openDevice(const char *devicePath) {
|
|
char buffer[80];
|
|
|
|
LOGV("Opening device: %s", devicePath);
|
|
|
|
AutoMutex _l(mLock);
|
|
|
|
int fd = open(devicePath, O_RDWR);
|
|
if(fd < 0) {
|
|
LOGE("could not open %s, %s\n", devicePath, strerror(errno));
|
|
return -1;
|
|
}
|
|
|
|
InputDeviceIdentifier identifier;
|
|
|
|
// Get device name.
|
|
if(ioctl(fd, EVIOCGNAME(sizeof(buffer) - 1), &buffer) < 1) {
|
|
//fprintf(stderr, "could not get device name for %s, %s\n", devicePath, strerror(errno));
|
|
} else {
|
|
buffer[sizeof(buffer) - 1] = '\0';
|
|
identifier.name.setTo(buffer);
|
|
}
|
|
|
|
// Check to see if the device is on our excluded list
|
|
List<String8>::iterator iter = mExcludedDevices.begin();
|
|
List<String8>::iterator end = mExcludedDevices.end();
|
|
for ( ; iter != end; iter++) {
|
|
const char* test = *iter;
|
|
if (identifier.name == test) {
|
|
LOGI("ignoring event id %s driver %s\n", devicePath, test);
|
|
close(fd);
|
|
return -1;
|
|
}
|
|
}
|
|
|
|
// Get device driver version.
|
|
int driverVersion;
|
|
if(ioctl(fd, EVIOCGVERSION, &driverVersion)) {
|
|
LOGE("could not get driver version for %s, %s\n", devicePath, strerror(errno));
|
|
close(fd);
|
|
return -1;
|
|
}
|
|
|
|
// Get device identifier.
|
|
struct input_id inputId;
|
|
if(ioctl(fd, EVIOCGID, &inputId)) {
|
|
LOGE("could not get device input id for %s, %s\n", devicePath, strerror(errno));
|
|
close(fd);
|
|
return -1;
|
|
}
|
|
identifier.bus = inputId.bustype;
|
|
identifier.product = inputId.product;
|
|
identifier.vendor = inputId.vendor;
|
|
identifier.version = inputId.version;
|
|
|
|
// Get device physical location.
|
|
if(ioctl(fd, EVIOCGPHYS(sizeof(buffer) - 1), &buffer) < 1) {
|
|
//fprintf(stderr, "could not get location for %s, %s\n", devicePath, strerror(errno));
|
|
} else {
|
|
buffer[sizeof(buffer) - 1] = '\0';
|
|
identifier.location.setTo(buffer);
|
|
}
|
|
|
|
// Get device unique id.
|
|
if(ioctl(fd, EVIOCGUNIQ(sizeof(buffer) - 1), &buffer) < 1) {
|
|
//fprintf(stderr, "could not get idstring for %s, %s\n", devicePath, strerror(errno));
|
|
} else {
|
|
buffer[sizeof(buffer) - 1] = '\0';
|
|
identifier.uniqueId.setTo(buffer);
|
|
}
|
|
|
|
// Make file descriptor non-blocking for use with poll().
|
|
if (fcntl(fd, F_SETFL, O_NONBLOCK)) {
|
|
LOGE("Error %d making device file descriptor non-blocking.", errno);
|
|
close(fd);
|
|
return -1;
|
|
}
|
|
|
|
// Allocate device. (The device object takes ownership of the fd at this point.)
|
|
int32_t deviceId = mNextDeviceId++;
|
|
Device* device = new Device(fd, deviceId, String8(devicePath), identifier);
|
|
|
|
#if 0
|
|
LOGI("add device %d: %s\n", deviceId, devicePath);
|
|
LOGI(" bus: %04x\n"
|
|
" vendor %04x\n"
|
|
" product %04x\n"
|
|
" version %04x\n",
|
|
identifier.bus, identifier.vendor, identifier.product, identifier.version);
|
|
LOGI(" name: \"%s\"\n", identifier.name.string());
|
|
LOGI(" location: \"%s\"\n", identifier.location.string());
|
|
LOGI(" unique id: \"%s\"\n", identifier.uniqueId.string());
|
|
LOGI(" driver: v%d.%d.%d\n",
|
|
driverVersion >> 16, (driverVersion >> 8) & 0xff, driverVersion & 0xff);
|
|
#endif
|
|
|
|
// Load the configuration file for the device.
|
|
loadConfiguration(device);
|
|
|
|
// Figure out the kinds of events the device reports.
|
|
|
|
uint8_t key_bitmask[sizeof_bit_array(KEY_MAX + 1)];
|
|
memset(key_bitmask, 0, sizeof(key_bitmask));
|
|
|
|
LOGV("Getting keys...");
|
|
if (ioctl(fd, EVIOCGBIT(EV_KEY, sizeof(key_bitmask)), key_bitmask) >= 0) {
|
|
//LOGI("MAP\n");
|
|
//for (int i = 0; i < sizeof(key_bitmask); i++) {
|
|
// LOGI("%d: 0x%02x\n", i, key_bitmask[i]);
|
|
//}
|
|
|
|
// See if this is a keyboard. Ignore everything in the button range except for
|
|
// gamepads which are also considered keyboards.
|
|
if (containsNonZeroByte(key_bitmask, 0, sizeof_bit_array(BTN_MISC))
|
|
|| containsNonZeroByte(key_bitmask, sizeof_bit_array(BTN_GAMEPAD),
|
|
sizeof_bit_array(BTN_DIGI))
|
|
|| containsNonZeroByte(key_bitmask, sizeof_bit_array(KEY_OK),
|
|
sizeof_bit_array(KEY_MAX + 1))) {
|
|
device->classes |= INPUT_DEVICE_CLASS_KEYBOARD;
|
|
|
|
device->keyBitmask = new uint8_t[sizeof(key_bitmask)];
|
|
if (device->keyBitmask != NULL) {
|
|
memcpy(device->keyBitmask, key_bitmask, sizeof(key_bitmask));
|
|
} else {
|
|
delete device;
|
|
LOGE("out of memory allocating key bitmask");
|
|
return -1;
|
|
}
|
|
}
|
|
}
|
|
|
|
// See if this is a trackball (or mouse).
|
|
if (test_bit(BTN_MOUSE, key_bitmask)) {
|
|
uint8_t rel_bitmask[sizeof_bit_array(REL_MAX + 1)];
|
|
memset(rel_bitmask, 0, sizeof(rel_bitmask));
|
|
LOGV("Getting relative controllers...");
|
|
if (ioctl(fd, EVIOCGBIT(EV_REL, sizeof(rel_bitmask)), rel_bitmask) >= 0) {
|
|
if (test_bit(REL_X, rel_bitmask) && test_bit(REL_Y, rel_bitmask)) {
|
|
device->classes |= INPUT_DEVICE_CLASS_TRACKBALL;
|
|
}
|
|
}
|
|
}
|
|
|
|
// See if this is a touch pad.
|
|
uint8_t abs_bitmask[sizeof_bit_array(ABS_MAX + 1)];
|
|
memset(abs_bitmask, 0, sizeof(abs_bitmask));
|
|
LOGV("Getting absolute controllers...");
|
|
if (ioctl(fd, EVIOCGBIT(EV_ABS, sizeof(abs_bitmask)), abs_bitmask) >= 0) {
|
|
// Is this a new modern multi-touch driver?
|
|
if (test_bit(ABS_MT_POSITION_X, abs_bitmask)
|
|
&& test_bit(ABS_MT_POSITION_Y, abs_bitmask)) {
|
|
device->classes |= INPUT_DEVICE_CLASS_TOUCHSCREEN | INPUT_DEVICE_CLASS_TOUCHSCREEN_MT;
|
|
|
|
// Is this an old style single-touch driver?
|
|
} else if (test_bit(BTN_TOUCH, key_bitmask)
|
|
&& test_bit(ABS_X, abs_bitmask) && test_bit(ABS_Y, abs_bitmask)) {
|
|
device->classes |= INPUT_DEVICE_CLASS_TOUCHSCREEN;
|
|
}
|
|
}
|
|
|
|
#ifdef EV_SW
|
|
// figure out the switches this device reports
|
|
uint8_t sw_bitmask[sizeof_bit_array(SW_MAX + 1)];
|
|
memset(sw_bitmask, 0, sizeof(sw_bitmask));
|
|
bool hasSwitches = false;
|
|
if (ioctl(fd, EVIOCGBIT(EV_SW, sizeof(sw_bitmask)), sw_bitmask) >= 0) {
|
|
for (int i=0; i<EV_SW; i++) {
|
|
//LOGI("Device %d sw %d: has=%d", device->id, i, test_bit(i, sw_bitmask));
|
|
if (test_bit(i, sw_bitmask)) {
|
|
hasSwitches = true;
|
|
if (mSwitches[i] == 0) {
|
|
mSwitches[i] = device->id;
|
|
}
|
|
}
|
|
}
|
|
}
|
|
if (hasSwitches) {
|
|
device->classes |= INPUT_DEVICE_CLASS_SWITCH;
|
|
}
|
|
#endif
|
|
|
|
if ((device->classes & INPUT_DEVICE_CLASS_TOUCHSCREEN)) {
|
|
// Load the virtual keys for the touch screen, if any.
|
|
// We do this now so that we can make sure to load the keymap if necessary.
|
|
status_t status = loadVirtualKeyMap(device);
|
|
if (!status) {
|
|
device->classes |= INPUT_DEVICE_CLASS_KEYBOARD;
|
|
}
|
|
}
|
|
|
|
if ((device->classes & INPUT_DEVICE_CLASS_KEYBOARD) != 0) {
|
|
// Load the keymap for the device.
|
|
status_t status = loadKeyMap(device);
|
|
|
|
// Set system properties for the keyboard.
|
|
setKeyboardProperties(device, false);
|
|
|
|
// Register the keyboard as a built-in keyboard if it is eligible.
|
|
if (!status
|
|
&& mBuiltInKeyboardId == -1
|
|
&& isEligibleBuiltInKeyboard(device->identifier,
|
|
device->configuration, &device->keyMap)) {
|
|
mBuiltInKeyboardId = device->id;
|
|
setKeyboardProperties(device, true);
|
|
}
|
|
|
|
// 'Q' key support = cheap test of whether this is an alpha-capable kbd
|
|
if (hasKeycodeLocked(device, AKEYCODE_Q)) {
|
|
device->classes |= INPUT_DEVICE_CLASS_ALPHAKEY;
|
|
}
|
|
|
|
// See if this device has a DPAD.
|
|
if (hasKeycodeLocked(device, AKEYCODE_DPAD_UP) &&
|
|
hasKeycodeLocked(device, AKEYCODE_DPAD_DOWN) &&
|
|
hasKeycodeLocked(device, AKEYCODE_DPAD_LEFT) &&
|
|
hasKeycodeLocked(device, AKEYCODE_DPAD_RIGHT) &&
|
|
hasKeycodeLocked(device, AKEYCODE_DPAD_CENTER)) {
|
|
device->classes |= INPUT_DEVICE_CLASS_DPAD;
|
|
}
|
|
|
|
// See if this device has a gamepad.
|
|
for (size_t i = 0; i < sizeof(GAMEPAD_KEYCODES)/sizeof(GAMEPAD_KEYCODES[0]); i++) {
|
|
if (hasKeycodeLocked(device, GAMEPAD_KEYCODES[i])) {
|
|
device->classes |= INPUT_DEVICE_CLASS_GAMEPAD;
|
|
break;
|
|
}
|
|
}
|
|
}
|
|
|
|
// If the device isn't recognized as something we handle, don't monitor it.
|
|
if (device->classes == 0) {
|
|
LOGV("Dropping device: id=%d, path='%s', name='%s'",
|
|
deviceId, devicePath, device->identifier.name.string());
|
|
delete device;
|
|
return -1;
|
|
}
|
|
|
|
LOGI("New device: id=%d, fd=%d, path='%s', name='%s', classes=0x%x, "
|
|
"configuration='%s', keyLayout='%s', keyCharacterMap='%s', builtinKeyboard=%s",
|
|
deviceId, fd, devicePath, device->identifier.name.string(),
|
|
device->classes,
|
|
device->configurationFile.string(),
|
|
device->keyMap.keyLayoutFile.string(),
|
|
device->keyMap.keyCharacterMapFile.string(),
|
|
toString(mBuiltInKeyboardId == deviceId));
|
|
|
|
struct pollfd pollfd;
|
|
pollfd.fd = fd;
|
|
pollfd.events = POLLIN;
|
|
pollfd.revents = 0;
|
|
mFds.push(pollfd);
|
|
mDevices.push(device);
|
|
|
|
device->next = mOpeningDevices;
|
|
mOpeningDevices = device;
|
|
return 0;
|
|
}
|
|
|
|
void EventHub::loadConfiguration(Device* device) {
|
|
device->configurationFile = getInputDeviceConfigurationFilePathByDeviceIdentifier(
|
|
device->identifier, INPUT_DEVICE_CONFIGURATION_FILE_TYPE_CONFIGURATION);
|
|
if (device->configurationFile.isEmpty()) {
|
|
LOGD("No input device configuration file found for device '%s'.",
|
|
device->identifier.name.string());
|
|
} else {
|
|
status_t status = PropertyMap::load(device->configurationFile,
|
|
&device->configuration);
|
|
if (status) {
|
|
LOGE("Error loading input device configuration file for device '%s'. "
|
|
"Using default configuration.",
|
|
device->identifier.name.string());
|
|
}
|
|
}
|
|
}
|
|
|
|
status_t EventHub::loadVirtualKeyMap(Device* device) {
|
|
// The virtual key map is supplied by the kernel as a system board property file.
|
|
String8 path;
|
|
path.append("/sys/board_properties/virtualkeys.");
|
|
path.append(device->identifier.name);
|
|
if (access(path.string(), R_OK)) {
|
|
return NAME_NOT_FOUND;
|
|
}
|
|
return VirtualKeyMap::load(path, &device->virtualKeyMap);
|
|
}
|
|
|
|
status_t EventHub::loadKeyMap(Device* device) {
|
|
return device->keyMap.load(device->identifier, device->configuration);
|
|
}
|
|
|
|
void EventHub::setKeyboardProperties(Device* device, bool builtInKeyboard) {
|
|
int32_t id = builtInKeyboard ? 0 : device->id;
|
|
android::setKeyboardProperties(id, device->identifier,
|
|
device->keyMap.keyLayoutFile, device->keyMap.keyCharacterMapFile);
|
|
}
|
|
|
|
void EventHub::clearKeyboardProperties(Device* device, bool builtInKeyboard) {
|
|
int32_t id = builtInKeyboard ? 0 : device->id;
|
|
android::clearKeyboardProperties(id);
|
|
}
|
|
|
|
bool EventHub::hasKeycodeLocked(Device* device, int keycode) const {
|
|
if (!device->keyMap.haveKeyLayout() || !device->keyBitmask) {
|
|
return false;
|
|
}
|
|
|
|
Vector<int32_t> scanCodes;
|
|
device->keyMap.keyLayoutMap->findScanCodes(keycode, &scanCodes);
|
|
const size_t N = scanCodes.size();
|
|
for (size_t i=0; i<N && i<=KEY_MAX; i++) {
|
|
int32_t sc = scanCodes.itemAt(i);
|
|
if (sc >= 0 && sc <= KEY_MAX && test_bit(sc, device->keyBitmask)) {
|
|
return true;
|
|
}
|
|
}
|
|
|
|
return false;
|
|
}
|
|
|
|
int EventHub::closeDevice(const char *devicePath) {
|
|
AutoMutex _l(mLock);
|
|
|
|
for (size_t i = FIRST_ACTUAL_DEVICE_INDEX; i < mDevices.size(); i++) {
|
|
Device* device = mDevices[i];
|
|
if (device->path == devicePath) {
|
|
LOGI("Removed device: path=%s name=%s id=%d fd=%d classes=0x%x\n",
|
|
device->path.string(), device->identifier.name.string(), device->id,
|
|
device->fd, device->classes);
|
|
|
|
#ifdef EV_SW
|
|
for (int j=0; j<EV_SW; j++) {
|
|
if (mSwitches[j] == device->id) {
|
|
mSwitches[j] = 0;
|
|
}
|
|
}
|
|
#endif
|
|
|
|
if (device->id == mBuiltInKeyboardId) {
|
|
LOGW("built-in keyboard device %s (id=%d) is closing! the apps will not like this",
|
|
device->path.string(), mBuiltInKeyboardId);
|
|
mBuiltInKeyboardId = -1;
|
|
clearKeyboardProperties(device, true);
|
|
}
|
|
clearKeyboardProperties(device, false);
|
|
|
|
mFds.removeAt(i);
|
|
mDevices.removeAt(i);
|
|
device->close();
|
|
|
|
device->next = mClosingDevices;
|
|
mClosingDevices = device;
|
|
return 0;
|
|
}
|
|
}
|
|
LOGE("remove device: %s not found\n", devicePath);
|
|
return -1;
|
|
}
|
|
|
|
int EventHub::readNotify(int nfd) {
|
|
#ifdef HAVE_INOTIFY
|
|
int res;
|
|
char devname[PATH_MAX];
|
|
char *filename;
|
|
char event_buf[512];
|
|
int event_size;
|
|
int event_pos = 0;
|
|
struct inotify_event *event;
|
|
|
|
LOGV("EventHub::readNotify nfd: %d\n", nfd);
|
|
res = read(nfd, event_buf, sizeof(event_buf));
|
|
if(res < (int)sizeof(*event)) {
|
|
if(errno == EINTR)
|
|
return 0;
|
|
LOGW("could not get event, %s\n", strerror(errno));
|
|
return 1;
|
|
}
|
|
//printf("got %d bytes of event information\n", res);
|
|
|
|
strcpy(devname, DEVICE_PATH);
|
|
filename = devname + strlen(devname);
|
|
*filename++ = '/';
|
|
|
|
while(res >= (int)sizeof(*event)) {
|
|
event = (struct inotify_event *)(event_buf + event_pos);
|
|
//printf("%d: %08x \"%s\"\n", event->wd, event->mask, event->len ? event->name : "");
|
|
if(event->len) {
|
|
strcpy(filename, event->name);
|
|
if(event->mask & IN_CREATE) {
|
|
openDevice(devname);
|
|
}
|
|
else {
|
|
closeDevice(devname);
|
|
}
|
|
}
|
|
event_size = sizeof(*event) + event->len;
|
|
res -= event_size;
|
|
event_pos += event_size;
|
|
}
|
|
#endif
|
|
return 0;
|
|
}
|
|
|
|
int EventHub::scanDir(const char *dirname)
|
|
{
|
|
char devname[PATH_MAX];
|
|
char *filename;
|
|
DIR *dir;
|
|
struct dirent *de;
|
|
dir = opendir(dirname);
|
|
if(dir == NULL)
|
|
return -1;
|
|
strcpy(devname, dirname);
|
|
filename = devname + strlen(devname);
|
|
*filename++ = '/';
|
|
while((de = readdir(dir))) {
|
|
if(de->d_name[0] == '.' &&
|
|
(de->d_name[1] == '\0' ||
|
|
(de->d_name[1] == '.' && de->d_name[2] == '\0')))
|
|
continue;
|
|
strcpy(filename, de->d_name);
|
|
openDevice(devname);
|
|
}
|
|
closedir(dir);
|
|
return 0;
|
|
}
|
|
|
|
void EventHub::dump(String8& dump) {
|
|
dump.append("Event Hub State:\n");
|
|
|
|
{ // acquire lock
|
|
AutoMutex _l(mLock);
|
|
|
|
dump.appendFormat(INDENT "BuiltInKeyboardId: %d\n", mBuiltInKeyboardId);
|
|
|
|
dump.append(INDENT "Devices:\n");
|
|
|
|
for (size_t i = FIRST_ACTUAL_DEVICE_INDEX; i < mDevices.size(); i++) {
|
|
const Device* device = mDevices[i];
|
|
if (device) {
|
|
if (mBuiltInKeyboardId == device->id) {
|
|
dump.appendFormat(INDENT2 "%d: %s (aka device 0 - built-in keyboard)\n",
|
|
device->id, device->identifier.name.string());
|
|
} else {
|
|
dump.appendFormat(INDENT2 "%d: %s\n", device->id,
|
|
device->identifier.name.string());
|
|
}
|
|
dump.appendFormat(INDENT3 "Classes: 0x%08x\n", device->classes);
|
|
dump.appendFormat(INDENT3 "Path: %s\n", device->path.string());
|
|
dump.appendFormat(INDENT3 "Location: %s\n", device->identifier.location.string());
|
|
dump.appendFormat(INDENT3 "UniqueId: %s\n", device->identifier.uniqueId.string());
|
|
dump.appendFormat(INDENT3 "Identifier: bus=0x%04x, vendor=0x%04x, "
|
|
"product=0x%04x, version=0x%04x\n",
|
|
device->identifier.bus, device->identifier.vendor,
|
|
device->identifier.product, device->identifier.version);
|
|
dump.appendFormat(INDENT3 "KeyLayoutFile: %s\n",
|
|
device->keyMap.keyLayoutFile.string());
|
|
dump.appendFormat(INDENT3 "KeyCharacterMapFile: %s\n",
|
|
device->keyMap.keyCharacterMapFile.string());
|
|
dump.appendFormat(INDENT3 "ConfigurationFile: %s\n",
|
|
device->configurationFile.string());
|
|
}
|
|
}
|
|
} // release lock
|
|
}
|
|
|
|
}; // namespace android
|