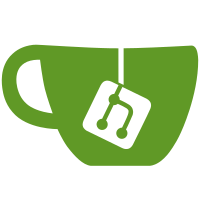
It is now possible to say: dumpsys SurfaceFlinger --latency to print latency information about all windows dumpsys SurfaceFlinger --latency window-name to print the latency stats of the specified window for instance: dumpsys SurfaceFlinger --latency SurfaceView The data consists of one line containing global stats, followed by 128 lines of tab separated timestamps in nanosecond. The first line currently contains the refresh period in nanosecond. Each 128 following line contains 3 timestamps, of respectively the app draw time, the vsync timestamp just prior the call to set and the timestamp of the call to set. Change-Id: Ib6b6da1d7e2e6ba49c282bdbc0b56a7dc203343a
134 lines
3.7 KiB
C++
134 lines
3.7 KiB
C++
/*
|
|
* Copyright (C) 2007 The Android Open Source Project
|
|
*
|
|
* Licensed under the Apache License, Version 2.0 (the "License");
|
|
* you may not use this file except in compliance with the License.
|
|
* You may obtain a copy of the License at
|
|
*
|
|
* http://www.apache.org/licenses/LICENSE-2.0
|
|
*
|
|
* Unless required by applicable law or agreed to in writing, software
|
|
* distributed under the License is distributed on an "AS IS" BASIS,
|
|
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
* See the License for the specific language governing permissions and
|
|
* limitations under the License.
|
|
*/
|
|
|
|
#ifndef ANDROID_DISPLAY_HARDWARE_H
|
|
#define ANDROID_DISPLAY_HARDWARE_H
|
|
|
|
#include <stdlib.h>
|
|
|
|
#include <ui/PixelFormat.h>
|
|
#include <ui/Region.h>
|
|
|
|
#include <GLES/gl.h>
|
|
#include <GLES/glext.h>
|
|
#include <EGL/egl.h>
|
|
#include <EGL/eglext.h>
|
|
|
|
#include <pixelflinger/pixelflinger.h>
|
|
|
|
#include "GLExtensions.h"
|
|
|
|
#include "DisplayHardware/DisplayHardwareBase.h"
|
|
#include "DisplayHardware/VSyncBarrier.h"
|
|
|
|
namespace android {
|
|
|
|
class FramebufferNativeWindow;
|
|
class HWComposer;
|
|
|
|
class DisplayHardware : public DisplayHardwareBase
|
|
{
|
|
public:
|
|
enum {
|
|
COPY_BITS_EXTENSION = 0x00000008,
|
|
BUFFER_PRESERVED = 0x00010000,
|
|
PARTIAL_UPDATES = 0x00020000, // video driver feature
|
|
SLOW_CONFIG = 0x00040000, // software
|
|
SWAP_RECTANGLE = 0x00080000,
|
|
};
|
|
|
|
DisplayHardware(
|
|
const sp<SurfaceFlinger>& flinger,
|
|
uint32_t displayIndex);
|
|
|
|
~DisplayHardware();
|
|
|
|
void releaseScreen() const;
|
|
void acquireScreen() const;
|
|
|
|
// Flip the front and back buffers if the back buffer is "dirty". Might
|
|
// be instantaneous, might involve copying the frame buffer around.
|
|
void flip(const Region& dirty) const;
|
|
|
|
float getDpiX() const;
|
|
float getDpiY() const;
|
|
float getRefreshRate() const;
|
|
float getDensity() const;
|
|
int getWidth() const;
|
|
int getHeight() const;
|
|
PixelFormat getFormat() const;
|
|
uint32_t getFlags() const;
|
|
void makeCurrent() const;
|
|
uint32_t getMaxTextureSize() const;
|
|
uint32_t getMaxViewportDims() const;
|
|
|
|
// waits for the next vsync and returns the timestamp of when it happened
|
|
nsecs_t waitForRefresh() const;
|
|
nsecs_t getRefreshPeriod() const;
|
|
nsecs_t getRefreshTimestamp() const;
|
|
|
|
uint32_t getPageFlipCount() const;
|
|
EGLDisplay getEGLDisplay() const { return mDisplay; }
|
|
|
|
void dump(String8& res) const;
|
|
|
|
// Hardware Composer
|
|
HWComposer& getHwComposer() const;
|
|
|
|
status_t compositionComplete() const;
|
|
|
|
Rect getBounds() const {
|
|
return Rect(mWidth, mHeight);
|
|
}
|
|
inline Rect bounds() const { return getBounds(); }
|
|
|
|
private:
|
|
void init(uint32_t displayIndex) __attribute__((noinline));
|
|
void fini() __attribute__((noinline));
|
|
int32_t getDelayToNextVSyncUs(nsecs_t* timestamp) const;
|
|
|
|
sp<SurfaceFlinger> mFlinger;
|
|
EGLDisplay mDisplay;
|
|
EGLSurface mSurface;
|
|
EGLContext mContext;
|
|
EGLConfig mConfig;
|
|
float mDpiX;
|
|
float mDpiY;
|
|
float mRefreshRate;
|
|
float mDensity;
|
|
int mWidth;
|
|
int mHeight;
|
|
PixelFormat mFormat;
|
|
uint32_t mFlags;
|
|
mutable uint32_t mPageFlipCount;
|
|
GLint mMaxViewportDims[2];
|
|
GLint mMaxTextureSize;
|
|
VSyncBarrier mVSync;
|
|
|
|
mutable Mutex mFakeVSyncMutex;
|
|
mutable nsecs_t mNextFakeVSync;
|
|
nsecs_t mRefreshPeriod;
|
|
mutable nsecs_t mLastHwVSync;
|
|
|
|
HWComposer* mHwc;
|
|
|
|
sp<FramebufferNativeWindow> mNativeWindow;
|
|
};
|
|
|
|
}; // namespace android
|
|
|
|
#endif // ANDROID_DISPLAY_HARDWARE_H
|