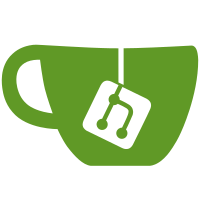
we need to clear the whole framebuffer in that situation because we can't trust the content of the FB when partial (fb preserving) updates are used. Bug: 5318492 Change-Id: I3f0e01b0fb665a34e44d88ad9f0f54a5d990060b
99 lines
2.5 KiB
C++
99 lines
2.5 KiB
C++
/*
|
|
* Copyright (C) 2010 The Android Open Source Project
|
|
*
|
|
* Licensed under the Apache License, Version 2.0 (the "License");
|
|
* you may not use this file except in compliance with the License.
|
|
* You may obtain a copy of the License at
|
|
*
|
|
* http://www.apache.org/licenses/LICENSE-2.0
|
|
*
|
|
* Unless required by applicable law or agreed to in writing, software
|
|
* distributed under the License is distributed on an "AS IS" BASIS,
|
|
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
* See the License for the specific language governing permissions and
|
|
* limitations under the License.
|
|
*/
|
|
|
|
#ifndef ANDROID_SF_HWCOMPOSER_H
|
|
#define ANDROID_SF_HWCOMPOSER_H
|
|
|
|
#include <stdint.h>
|
|
#include <sys/types.h>
|
|
|
|
#include <EGL/egl.h>
|
|
|
|
#include <hardware/hwcomposer.h>
|
|
|
|
#include <utils/StrongPointer.h>
|
|
#include <utils/Vector.h>
|
|
|
|
namespace android {
|
|
// ---------------------------------------------------------------------------
|
|
|
|
class String8;
|
|
class SurfaceFlinger;
|
|
class LayerBase;
|
|
|
|
class HWComposer
|
|
{
|
|
public:
|
|
|
|
HWComposer(const sp<SurfaceFlinger>& flinger);
|
|
~HWComposer();
|
|
|
|
status_t initCheck() const;
|
|
|
|
// tells the HAL what the framebuffer is
|
|
void setFrameBuffer(EGLDisplay dpy, EGLSurface sur);
|
|
|
|
// create a work list for numLayers layer
|
|
status_t createWorkList(size_t numLayers);
|
|
|
|
// Asks the HAL what it can do
|
|
status_t prepare() const;
|
|
|
|
// disable hwc until next createWorkList
|
|
status_t disable();
|
|
|
|
// commits the list
|
|
status_t commit() const;
|
|
|
|
// release hardware resources
|
|
status_t release() const;
|
|
|
|
size_t getNumLayers() const;
|
|
hwc_layer_t* getLayers() const;
|
|
|
|
// updated in preapre()
|
|
size_t getLayerCount(int type) const;
|
|
|
|
// for debugging
|
|
void dump(String8& out, char* scratch, size_t SIZE,
|
|
const Vector< sp<LayerBase> >& visibleLayersSortedByZ) const;
|
|
|
|
private:
|
|
struct cb_context {
|
|
hwc_procs_t procs;
|
|
HWComposer* hwc;
|
|
};
|
|
static void hook_invalidate(struct hwc_procs* procs);
|
|
void invalidate();
|
|
|
|
sp<SurfaceFlinger> mFlinger;
|
|
hw_module_t const* mModule;
|
|
hwc_composer_device_t* mHwc;
|
|
hwc_layer_list_t* mList;
|
|
size_t mCapacity;
|
|
mutable size_t mNumOVLayers;
|
|
mutable size_t mNumFBLayers;
|
|
hwc_display_t mDpy;
|
|
hwc_surface_t mSur;
|
|
cb_context mCBContext;
|
|
};
|
|
|
|
|
|
// ---------------------------------------------------------------------------
|
|
}; // namespace android
|
|
|
|
#endif // ANDROID_SF_HWCOMPOSER_H
|